Interface Vs Abstract Class In Java
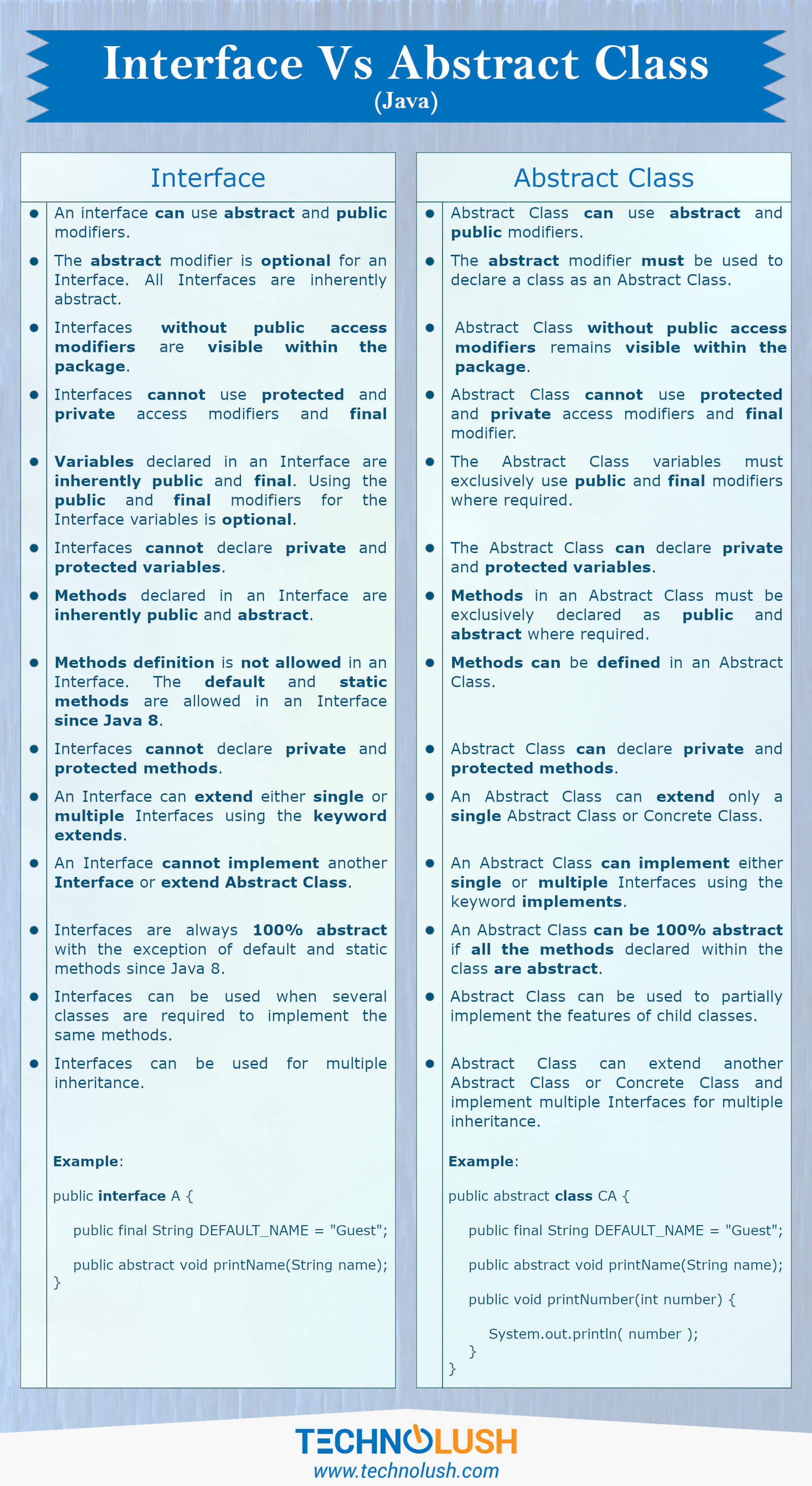
Interface Vs Abstract Class
Java is among the most popular Object-Oriented Programming (OOP) languages and widely used by enterprises to build reliable, secure, and maintainable software. Interfaces and Classes are the most important aspect of any OOP language and considered as the base for designing good software that can be easily understood, extended and managed by others. This tutorial provides a list of differences between an Interface and Abstract Class. You can also refer Interface Vs Abstract Class In Java for example.
Interface | Abstract Class |
---|---|
An interface can use abstract and public modifiers. | Abstract Class can use abstract and public modifiers. |
The abstract modifier is optional for an Interface. All Interfaces are inherently abstract. | The abstract modifier must be used to declare a class as an Abstract Class. |
Interfaces without public access modifiers are visible within the package. | Abstract Class without public access modifiers remains visible within the package. |
Interfaces cannot use protected and private access modifiers and final modifier. | Abstract Class cannot use protected and private access modifiers and final modifier. |
Variables declared in an Interface are inherently public and final. Using the public and final modifiers for the Interface variables is optional. | The Abstract Class variables must exclusively use public and final modifiers where required. |
Interfaces cannot declare private and protected variables. | The Abstract Class can declare private and protected variables. |
Methods declared in an Interface are inherently public and abstract. | Methods in an Abstract Class must be exclusively declared as public and abstract where required. |
Methods definition is not allowed in an Interface. The default and static methods are allowed in an Interface since Java 8. | Methods can be defined in an Abstract Class. |
Interfaces cannot declare private and protected methods. | Abstract Class can declare private and protected methods. |
An Interface can extend either single or multiple Interfaces using the keyword extends. | An Abstract Class can extend only a single Abstract Class or Concrete Class. |
An Interface cannot implement another Interface or extend Abstract Class. | An Abstract Class can implement either single or multiple Interfaces using the keyword implements. |
Interfaces are always 100% abstract with the exception of default and static methods since Java 8. | An Abstract Class can be 100% abstract if all the methods declared within the class are abstract. |
Interfaces can be used when several classes are required to implement the same methods. | Abstract Class can be used to partially implement the features of child classes. |
Interfaces can be used for multiple inheritance. | Abstract Class can extend another Abstract Class or Concrete Class and implement multiple Interfaces for multiple inheritance. |
Interface Example
public interface A {
public final String DEFAULT_NAME = "Guest";
public abstract void printName(String name);
}
Abstract Class Example
public abstract class CA {
public final String DEFAULT_NAME = "Guest";
public abstract void printName(String name);
public void printNumber(int number) {
System.out.println( number );
}
}
Write a Comment
Discussion Forum by DISQUS