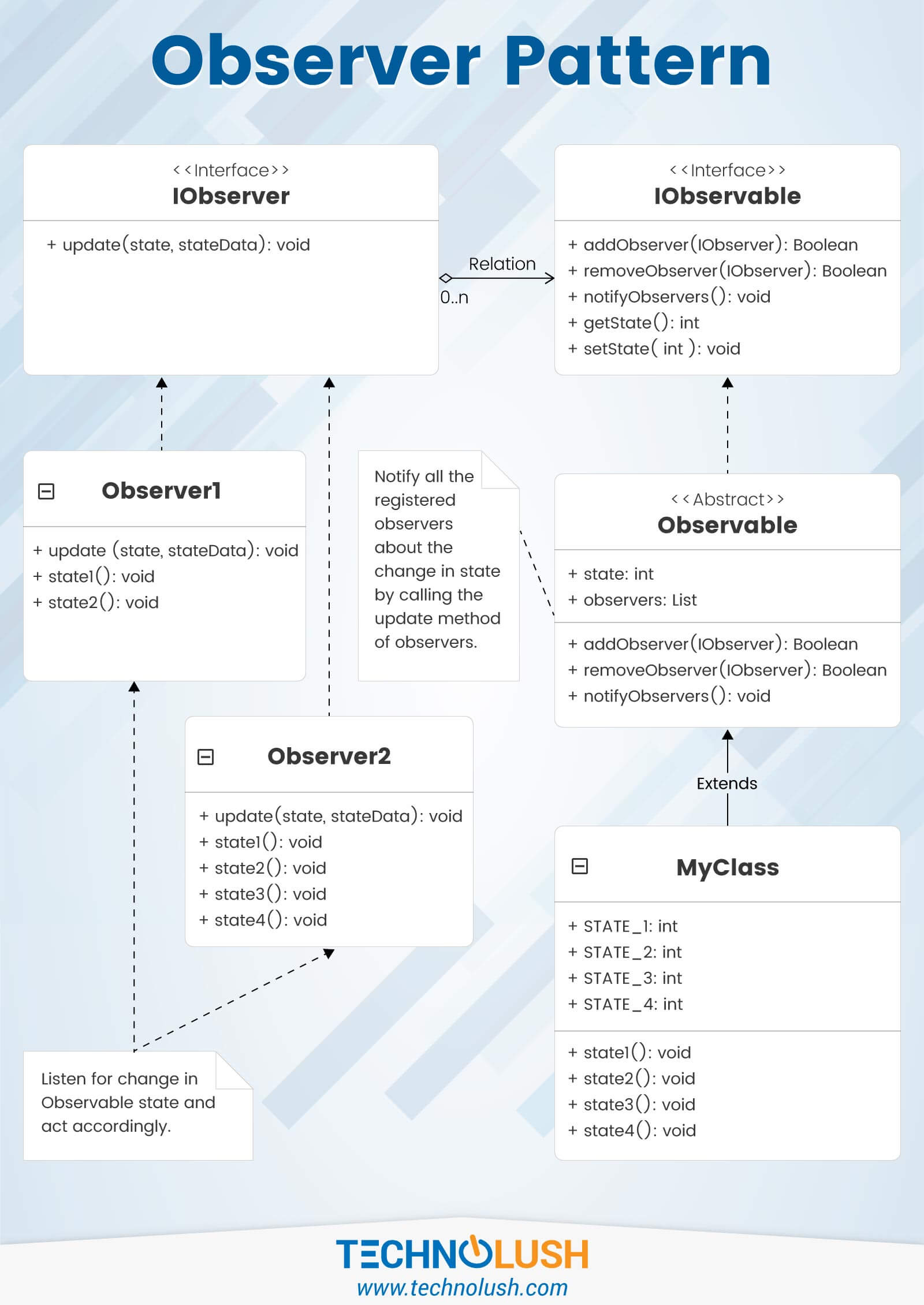
In this post, we will be discussing the very famous pattern i.e. Observer Pattern in which a subject distributes the state data to the registered listeners also called as observers. The actual implementation might vary based on the scenario, but the basic concepts to implement the Observer Pattern remains the same.
Subject
In the Observer Pattern, the Subject maintains the state and a list of all the observers listening to it. When there is a change in the state or updates available specific to a state, the Subject i.e. Observable distributes the event by calling all the listeners or observers available in its list.
It can also be implemented in the simplest way where it only needs to call the observer's update method without maintaining any kind of state or state data.
Observer
The Observer wait for any change in state or updated state data distributed by the Subject in order to take the corresponding action.
How Does It Work?
The main program has to maintain references to the Subject Or Observable, Listeners or Observers and it must also provide the way to register or unregister the listeners or observers.
The main program will trigger the various states and state change of the Subject and the same will be propagated by the Subject to it's registered listeners. The listeners will further take the appropriate action based on the event and event data passed by the Subject.
Practical Examples
In the previous sections, we have discussed the basic concepts specific to the Observer Pattern. In this section, we will discuss the same with some real-time examples as listed below.
Cricket Scoreboard
The main program maintains the references to the score feed using the subject implemented as ScoreFeeder. The ScoreFeeder gets the score data from some external interface or APIs and acts as a distributor to distribute the live feed to all the registered observers.
The main program also maintains references to the LiveScoreCard, ScoreRecorder, and ScorePredictor listening to the latest score data. There won't be any direct relation between the ScoreFeeder and all the listeners to have a loose coupling between them. This makes the ScoreFeeder add more listeners in the future without changing it.
The LiveScoreCard will simply update the score screen and show the latest score data for display purpose.
The ScoreRecorder records the score data so that some analytics and algorithms can be implemented on the recorded data.
The ScorePredictor will update the screen section specific for the score prediction utilizing the data recorded by the ScoreRecorder. It will also show the most recent state change or state update.
News Feed
In this example, we can have a very simple implementation of the Observer Pattern where the main class maintains references to the NewsFeed implemented as Subject and the NewsScreen as the listener.
The NewsFeed gets the latest need via external interface or APIs and simply pass the news to its listeners by calling their update method. The NewsScreen will simply show the most recent news on the screen.
These are some of the practical usages of the Observer Pattern.
Number Converter
It's another simple example in which the main program will maintain references to the NumberFeed implemented as the Subject or Observable. The NumberFeed will get a number from the external interface and pass the same to the registered listeners.
The possible observers could be BinaryNumber, OctalNumber, and HexadecimalNumber where each of these observers changes a specific section of the screen to display the same number feed in a different format.