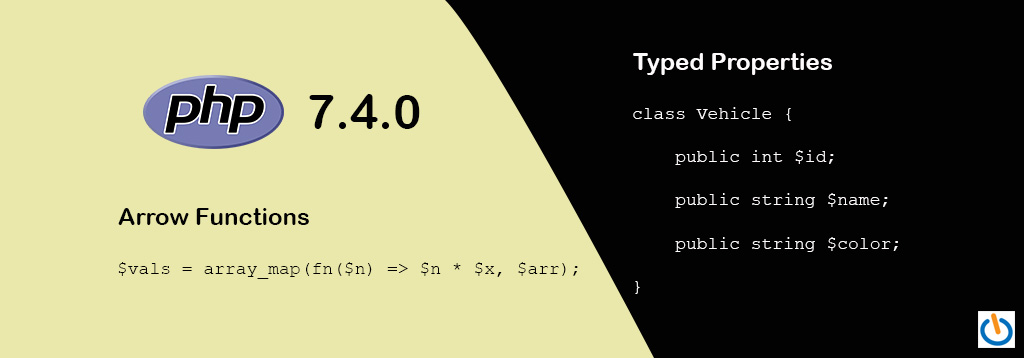
With the release of PHP 7.4, there are several new features and enhancements available in PHP. This post provides a list of the new features and enhancements introduced in PHP 7.4.
The new features and enhancements introduced in PHP 7.4 are listed below.
PHP Core
This section covers the new features and enhancements available in PHP Core since PHP 7.4.
Typed Properties
Before version 7, PHP was considered as the weakly typed language. As PHP is moving forward, it's focusing more on type hinting. Now we can define the type of properties as shown in the below-mentioned example. It makes it much easier to identify the type of values that a variable can have. You can explore typed properties in more detail by following Typed Properties In PHP.
class Vehicle {
public int $id;
public string $name;
public string $color;
}
Arrow Functions
Arrow functions also called short closures can be used to return implicit value as shown below.
// Perform calculation
$values = array_map(fn($num) => $num * $factor, $arr);
// Properties list
$names = array_map(fn(Car $car) => $car->name, $cars);
// Properties list with type hinting
$names = array_map(fn(Car $car): string => $car->name, $cars);
In the first example, the array elements of the array $arr will be multiplied by the given $factor to return the updated values. In the second example, the array_map will return an array of cars names.
Limited return type covariance and argument type contravariance
Covariant Return Types - This feature is relevant to inheritance. Now the child classes can change the return type of an overridden function. The return type must be the child class as compared to the return type of parent class and pass the IS-A relationship to be considered as a valid return type. The below-mentioned example further clears it.
class A {
public int $id;
public string $name;
public function __construct(int $id, string $name) {
$this->id = $id;
$this->name = $name;
}
}
class B extends class A {
}
class C {
public function generate(int $id, string $name): A {
return new A($id, $name);
}
}
class D extends C {
public function generate(int $id, string $name): B {
return new B($id, $name);
}
}
We can see that the return type of the overridden function generate is different in class D as compared to the generate function class C, but at the same time, the return type of the overridden function is a child type of the parent function. The return type of the overridden function must pass the IS-A relationship test.
Contravariant Argument Types - This feature is also relevant to inheritance. The argument type of the overridden function in the child class must be the parent class as shown in the below-mentioned example.
class A {
public int $id;
public string $name;
public function __construct(int $id, string $name) {
$this->id = $id;
$this->name = $name;
}
}
class B extends class A {
}
class C {
public function process(B $arg) {
// Process here
}
}
class D extends C {
public function process(A $arg) {
// Process here
}
}
We can see that the argument type of the overridden function process is different in class D as compared to the process function class C, but at the same time, the argument type of the overridden function is a parent type of the parent function. The argument type of the parent class function must pass the IS-A relationship test.
Null coalescing assignment operator
PHP 7.4 introduces a shorthand for null coalescing operations as shown below.
// $num takes the default value as 10 in case $num is empty
$num = $num ?? 10;
// PHP 7.4 - Shorthand notation of the above code
$num ??= 10;
Unpacking inside arrays
PHP 7.4 allows the spread operator in arrays having numerical keys as shown below.
$arr1 = [50, 58, 12, 72];
$arr2 = [15, 8, 10];
$result = [1, ...$arr1, 8, ...$arr2];
// Output - The $result will be
//[1, 50, 58, 12, 72, 8, 15, 8, 10]
The same is applicable for associative arrays having numerical keys.
Numeric literal separator
PHP 7.4 allows using underscores to separate digits of numeric literals as shown below.
$num = 120_225.12;
echo $num;
// Output
120225.12
Weak references
Weak references do not prevent the objects from being destroyed. Normally, any object referenced by another object won't be considered for collection by the garbage collector.
Definition from PHP Wiki - A weak reference provides a reference to an object that does not prevent it from being collected by the garbage collector (GC) as opposed from a strong reference (a normal variable containing an object instance).
Allow exceptions from __toString()
PHP 7.4 allows exceptions to be thrown in the function __toString.
CURL
The CURL features and enhancements introduced in PHP 7.4 are listed below.
Stream Wrappers In CURLFile
CURLFile supports stream wrappers in addition to plain file names. The extension must be built against libcurl >= 7.56.0.
Filter
Min and Max Range in FILTER_VALIDATE_FLOAT
The filter FILTER_VALIDATE_FLOAT supports the min_range and max_range options.
FFI
FFI Extension
Foreign Function Interface(FFI) is a new extension added in PHP 7.4. It can be used to call native functions, access native variables, and create/access data structures defined in C libraries.
GD
Scatter Image Filter
A new image filter i.e. IMG_FILTER_SCATTER has been added to apply a scatter filter to images.
Hash
CRC32 Hash
PHP 7.4 added crc32c hash using Castagnoli's polynomial.
Multibyte String
Added mb_str_split() function
The newly added function mb_str_split works similar to the function str_split, but on multi-byte strings.
Opcache Preloading
Opcache Preloading
PHP 7.4 added support for preloading code to avoid multiple loading of the files for each request. The files will be available in memory for subsequent requests to speed up request processing.
PDO
Username and Password in PDO DSN
We can specify the username and password as part of the PDO DSN. This feature is available for popular database drivers including mysql, mssql, sybase, dblib, firebird, and oci.
Extensions Removed
Some of the extensions have been moved to PECL and are no more part of the PHP distribution. These extensions include Firebird/Interbase, Recode, and WDDX.
New Classes & Interfaces
A new class ReflectionReference is introduced in PHP 7.4.
New Functions
Several new functions are introduced in PHP 7.4 including get_mangled_object_vars(), password_algos(), and sapi_windows_set_ctrl_handler(). You can follow the official PHP Site to get the complete list of the new functions.
Features Deprecated in PHP 7.4
There are several features marked as deprecated in PHP 7.4.
Nested ternary operators without explicit parentheses
The nested ternary operators without using the parentheses have been deprecated in PHP 7.4. One must start using parentheses for nested ternary operators as shown below.
$num1 = 10;
$num2 = 20;
// Deprecated
$num = $num1 > 0 ? $num1 : $num2 > 0 ? $num2 : 0;
// OK
$num = $num1 > 0 ? $num1 : ( $num2 > 0 ? $num2 : 0);
Array and string offset access using curly braces
Accessing the array and string offset using curly braces is deprecated in PHP 7.4 as shown below.
$arr = [12, 25, 45, 98];
// Deprecated
$val = $arr{2};
// OK
$val = $arr[2];
You can also follow the official PHP Site to know more about the features deprecated in PHP 7.4.